Bring your own app
Webflow Cloud deploys your app using Cloudflare Workers on the Edge runtime, enabling fast, globally distributed hosting. Before deploying to Webflow Cloud, your project may require some configuration to ensure compatibility with the Edge environment.
In this guide, you’ll learn how to create projects and environments, configure your app for Webflow Cloud, and deploy your app to your Webflow site,
Get Started with Webflow Cloud
To familiarize yourself with Webflow Cloud, it’s recommended to create your first Webflow Cloud project from the Webflow CLI before configuring a custom project.
Time Estimate: 30 minutes
Prerequisites:
- A Webflow account
- A Webflow site with components
- A GitHub account
- One of the following:
- An Astro project
- A Next.js project (version 15 or higher)
- Node.js 20.0.0 or higher and
npm
installed- Note: Currently, Webflow Cloud only supports using the
npm
package manager
- Note: Currently, Webflow Cloud only supports using the
Webflow Cloud is currently in beta
Webflow Cloud is currently in beta. Please see webflow.com/cloud for access.
1. Create a new Webflow Cloud project
Connect GitHub to Webflow Cloud, create a project, and configure an environment for automated deployments.
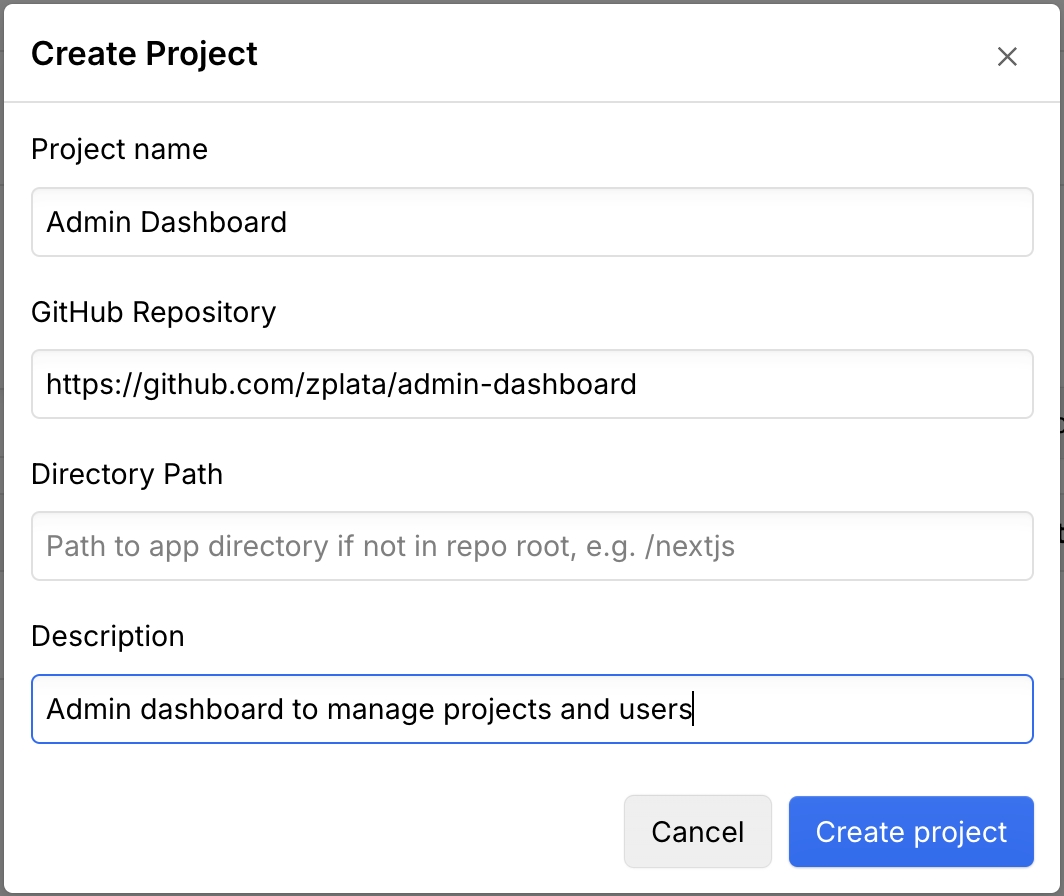
Open Webflow Cloud
In Webflow, navigate to your site’s settings and select “Webflow Cloud” from the sidebar.
Authenticate with Github
Click the “Login to GitHub” button to connect your GitHub account. Then click the “Install GitHub” button. Follow the instructions to allow Webflow Cloud to access your GitHub repositories.
Add project details
- Choose a name for your Webflow Cloud project.
- Provide the URL of your newly created GitHub repository.
- Optionally, enter a description for your app.
- Click “Create project” to save your project.
2. Configure your project for Webflow Cloud
Webflow Cloud leverages Cloudflare Workers on the Edge runtime to deploy your applications. The Edge runtime environment differs from traditional Node.js servers, requiring specific configurations for compatibility.
The following steps outline common migration patterns to help you adapt your application for Webflow Cloud. Note that depending on your application’s specific requirements, you may need to make additional adjustments beyond these configurations.
Next.js
Astro
Webflow Cloud is compatible with Next.js 15 and higher
Webflow Cloud is compatible with Next.js 15 and higher. If you’re using a version of Next.js lower than 15, please upgrade to the latest version.
Configure your base path
In Webflow Cloud, your application is served from the mount path you configured in your environment settings. For example, if your mount path is /app
, your application will be accessible at yourdomain.webflow.io/app
. To ensure proper routing and asset loading, you must configure the basePath
and assetPrefix
properties in your next.config.ts
file to match this mount path exactly.
Install and configure OpenNext
OpenNext is an adapter designed specifically for deploying Next.js applications to cloud environments like Webflow Cloud. By using OpenNext, you can deploy your Next.js app without managing complex infrastructure configurations yourself.
-
Install OpenNext
In your terminal, navigate to your project and run the following command to install OpenNext: -
Configure OpenNext
Create a new configuration file namedopen-next.config.ts
in your project’s root directory. This file configures OpenNext to work with Webflow Cloud’s deployment environment.open-next.config.ts
Set up local testing for Webflow Cloud
Webflow Cloud uses Wrangler - Cloudflare’s CLI tool - to bridge the gap between local development and cloud deployment. By integrating Wrangler into your workflow, you can identify and resolve compatibility issues early, significantly reducing debugging time after deployment.
-
Install Wrangler
To get started, install and configure Wrangler as a dev dependency in your project. -
Set up your Wrangler configuration
Create awrangler.jsonc
file in your project root that defines how your application will run in development.wrangler.jsoncSee the Cloudflare documentation for more details on how to configure Wrangler.
-
Create a Cloudflare environment file
Create a new file namedcloudflare-env.d.ts
in your project’s root directory. This file will allow you to use environment variables defined in your Webflow Cloud environment.cloudflare-env.d.ts -
Add the development preview command
Add this script to yourpackage.json
file to enable local testing with Wrangler. This command builds your Next.js app and immediately serves it using the Edge runtime, giving you an exact preview of how your app will perform in production on Webflow Cloud:package.json
3. Manage assets and APIs
Next.js
Astro
Asset references
When deployed to Webflow Cloud, all your static assets must include the configured mount path to load correctly. Without this path prefix, browsers will request assets from the root domain, resulting in 404 errors.
We recommend constructing asset paths using assetPrefix
. Webflow Cloud will automatically configure a CDN for assets that use assetPrefix
for improved loading performance.
Implement this pattern in your components for reliable asset loading:
APIs
When using Next.js with a configured base path, there’s an important distinction between API route definitions and client-side requests:
- Server-side API route handlers are automatically mounted at your base path by Next.js. To ensure your API routes run on the Edge runtime, add the
export const runtime = 'edge';
directive to your API route. - Client-side fetch calls must manually include the base path to correctly reach your endpoints
Without these adjustments, your API routes will not build properly and your client-side fetch calls will fail by targeting the wrong URL. Implement these patterns in all your APIs and client-side data fetching functions:
Edge Runtime: Use `fetch` API
The Edge runtime has limited API support. Stick to fetch
for API calls and avoid third-party clients like axios
which may not be compatible.
4. Configure environment variables
Access environment variable settings
In Webflow Cloud, navigate to your project’s environment settings:
- Click your project name in the dashboard
- Select the specific environment you want to configure
- Click the “Environment Variables” tab
Add and configure environment variables
Add each environment variable that your application requires:
- Click “Add Variable”
- Enter a descriptive “Variable Name” (e.g.,
DATABASE_URL
,API_KEY
) - Enter the corresponding “Variable Value”
- Toggle “Secret” for sensitive values that should be encrypted (API keys, tokens, etc.)
- Click “Add Variable” to save
Repeat this process for all required variables.
Environment variables are only available at runtime
Environment variables in Webflow Cloud are injected at runtime only and are not accessible during the build process. Keep the following in mind to avoid build failures:
- Do not include environment variable validation or required checks that run during build time
- Use conditional logic to handle cases where environment variables might be undefined during builds
5. Deploy your project
After configuring your project:
-
Test your app in the local preview environment
Use the local preview environment with Wrangler to simulate the same base path configuration as your production environment. -
Deploy using the Webflow CLI
In your terminal, run the following command to deploy your project to Webflow Cloud:Additionally, when you commit your changes to your GitHub branch, Webflow Cloud will automatically detect the changes and deploy your project to your environment. Learn more about deployments in the documentation.
Your deployment may take up to 2 minutes to complete
View your deployment in the “Environment Details” dashboard. Review the status of your deployment by viewing the build logs.
-
View your app at your site’s URL + mount path
Once your app has been successfully deployed, navigate to your site’s domain and mount path to see your newly deployed Webflow Cloud app!
🎉Congratulations!
You’ve successfully deployed your app on Webflow Cloud.
Next steps
Now that you’ve successfully deployed your app on Webflow Cloud, here’s what you can do next.
Learn how to use DevLink to sync your Webflow styles, variables, and components with your app
Learn how to manage environments for different stages of development
Explore deployment options and Webflow Cloud’s CI/CD integration with GitHub to streamline your release process
Review the limits for Webflow Cloud
Troubleshooting
I'm seeing a 404 error when I try to access my app
After creating a new environment, you’ll need to publish your Webflow site to make your environment live.
A deployment isn't kicking off when I push to my Github repo
The Webflow Cloud GitHub App may not have access to your repository. To check, go to the Webflow Cloud
tab in your Webflow site settings and click “Install GitHub App.” Follow the prompts on GitHub to ensure Webflow has access to read from your repository. Once you grant access, try committing to the branch that Webflow Cloud should be monitoring for deployments in your app.
My assets or API routes aren't loading correctly
Check that you’re correctly using the base path in all asset and API references. Look for fixed paths that might be missing the base path prefix.
Authentication isn't working properly
Verify that your callback URLs include the correct base path and that you’re not duplicating the base path in your code references.
My build is failing in Webflow Cloud
Check your project’s build logs in the Webflow Cloud dashboard. Common issues include:
- Incompatible Node.js version
- Environment variables not configured correctly
- Missing or incorrect framework configuration in the following files:
webflow.json
next.config.js
orAstro.config.js
wrangler.jsonc
cloudflare-env.d.ts
orworker-configuration.d.ts
- Custom build commands not supported (Webflow Cloud only uses
Astro build
ornext build
)