Styling and Interactions
This guide will help you understand how DevLink preserves the styles of your design system and the interactions of your Webflow components in your app.
Styles
Add your global styles to your application to ensure that your Webflow components are styled consistently across your app.
DevLink uses a combination of CSS Modules and global styles to maintain the look and feel of your Webflow components:
CSS Modules for component styles
Each component exported through DevLink gets its own CSS Module file with component-specific styles that:
- Isolate component styles to prevent global CSS conflicts
- Preserve Webflow styling details including media queries
- Maintain responsive behavior
- Support special selectors like
:global()
for Webflow classes
When working with custom HTML IDs in components, see the Custom IDs and selectors section below.
Global styles
The global.css
file contains styles that apply across all components:
- Normalize.css resets for browser consistency
- Webflow’s base styles (e.g.
.w-slider
and.w-nav-link
) - Font imports from your Webflow project
- CSS Variables for colors and other properties
- Default element styling and form element resets
- Custom CSS tag selectors from your site (e.g. “All Links” or “Body (All Pages)” style selectors)
For global styling that should affect all components, use the “Body (all pages)” tag selector in Webflow rather than setting classes directly on the body element, as the latter won’t be included in global.css
.
Advanced configuration
If you need to customize the styles of your Webflow components, you can configure the following options:
-
CSS modules
By default, DevLink uses CSS modules to isolate component styles. If needed, you can disable this with:.webflowrc.js -
Tag selectors
To minimize global styling and only include the bare essentials:.webflowrc.jsWhen using
skipTagSelectors: true
, ensure parent elements of DevLink components have defined heights/widths, or setheight: 100%
on your HTML and body elements to allow proper rendering of navigation components.
Interactions
Webflow interactions are JavaScript-powered animations and transitions. DevLink preserves these interactions by wrapping them in a special provider component:
The DevLinkProvider must wrap your application for Webflow interactions to work. Webflow Cloud apps can handle this automatically if you set provider: true
in your DevLink configuration.
Page interactions
Webflow components support page triggers with a limitation: DevLink only exports the first page interaction. If a component uses multiple page interactions across different pages, DevLink will only export the first one.
Advanced styling
Custom IDs and selectors
When you add custom HTML IDs to your Webflow components, DevLink automatically transforms them using CSS Modules. This transformation helps keep your component styles organized and prevents any conflicts between different parts of your application. The transformed IDs follow a specific pattern that combines your component name with your custom ID:
<ComponentName>_<custom-id>__<unique-identifier>
For example, if you add a custom ID to a Grid element within a Hero
component named featured-section
, DevLink will transform it into a unique identifier like Hero_featured-section__abc123
in the generated code.
To target these elements with CSS, use wildcard selectors that match the component name and custom ID pattern:
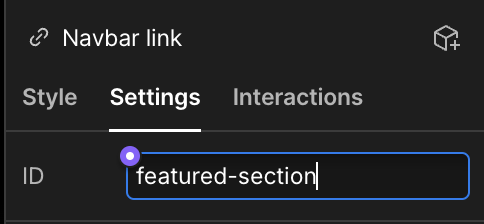
Dynamic IDs and styling hooks
Some Webflow elements use HTML IDs as styling hooks, including Grid and Quick Stack components. These IDs are visually indicated by pink markers in the Style Panel.
When attempting to make these IDs dynamic using component properties, you’ll encounter a technical limitation. DevLink can’t simultaneously preserve the required CSS styling references while supporting dynamic ID values. Here’s why:
The issue arises because Webflow generates CSS that directly references these IDs:
When you make the ID dynamic, the CSS selector no longer matches, breaking the styling.
Recommended solution: Custom attributes
Instead of modifying IDs, use custom attributes for dynamic values. This preserves Webflow’s styling while allowing for dynamic identification: