Getting Started
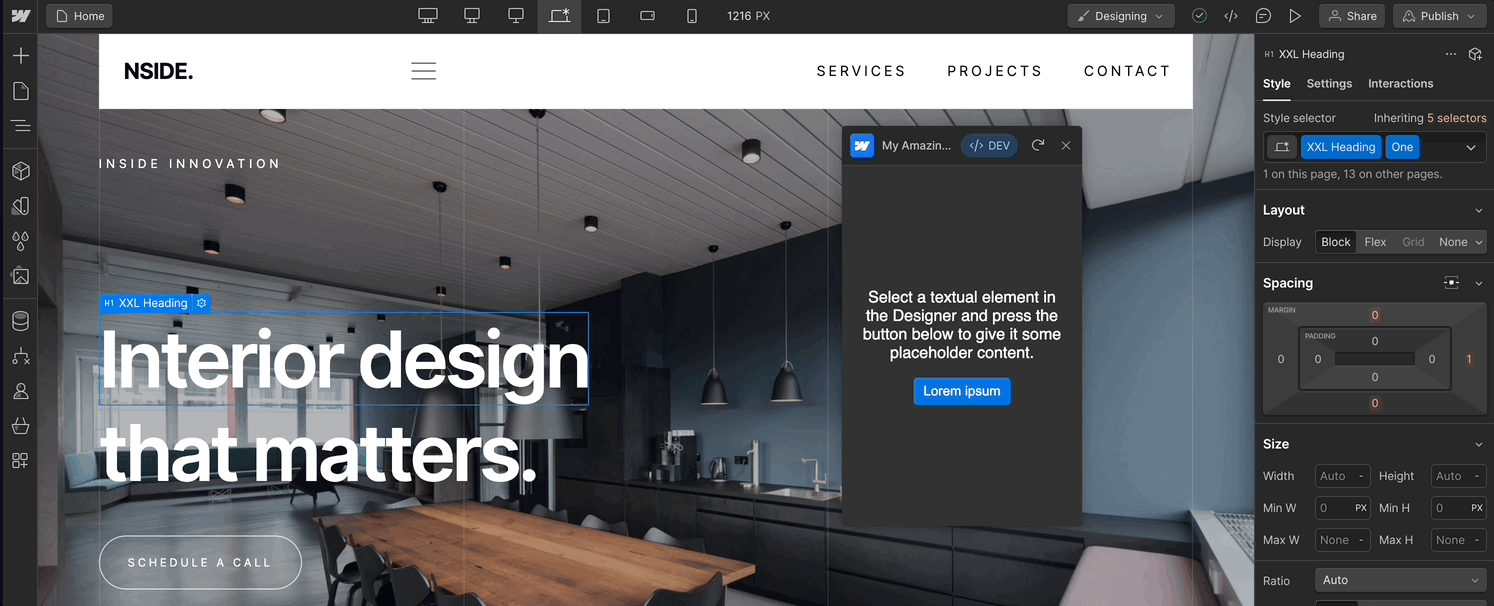
This guide will walk you through the setup of a basic Designer Extension replaces the text of an element with “Lorem Ipsum.” By the end of this guide, you’ll have completed the following steps:
- Install the Webflow CLI
- Initialize a Designer Extension starter project
- Run a Designer Extension in the Webflow Designer
- Update Elements on a page
- Modify Designer Extension with the Designer APIs
Prerequisites
To successfully follow along with this guide, please ensure your system meets the following requirements and you have the following resources:
- Node.js 16.20 or later
- A Webflow site for development and testing
- A registered Webflow App that’s installed to your test site. If you haven’t set up an App yet, please follow our guide on creating an App.