Designer Extensions
Designer Extensions offer a powerful way to expand the functionality of the Designer. You can now create tools to help Visual Designers as they’re adding designs and functionality to their sites. But, before we jump into their development, let’s walk through how Designer Extensions work.
Designer Extensions are single-page applications that are loaded via an iframe in the Webflow Designer. This iframe can host a simple script that uses the new client-side Designer APIs, or a more complex web app that connects to a backend server and other third-party services.
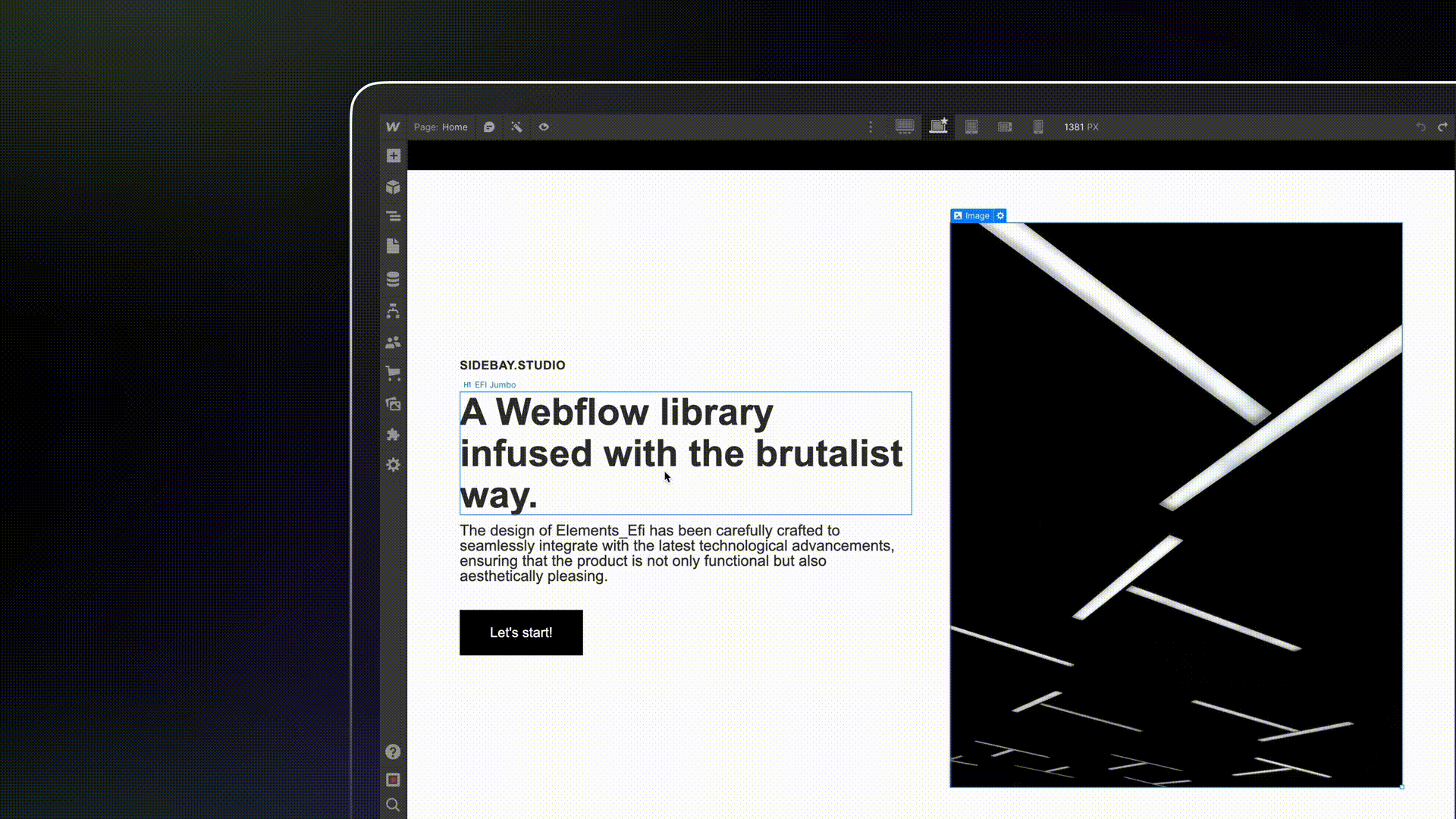
Designer APIs
Designer APIs allow Apps to manage elements, properties, text, and styles on the active Canvas. These APIs interact with the browser to access and manipulate elements; handle user interactions; and retrieve data from external sources. When an App modifies an Element on the Canvas — for example, updating the padding — this change will appear to have been made directly by the user during their browser session, and will be recorded in the user's undo/redo history.
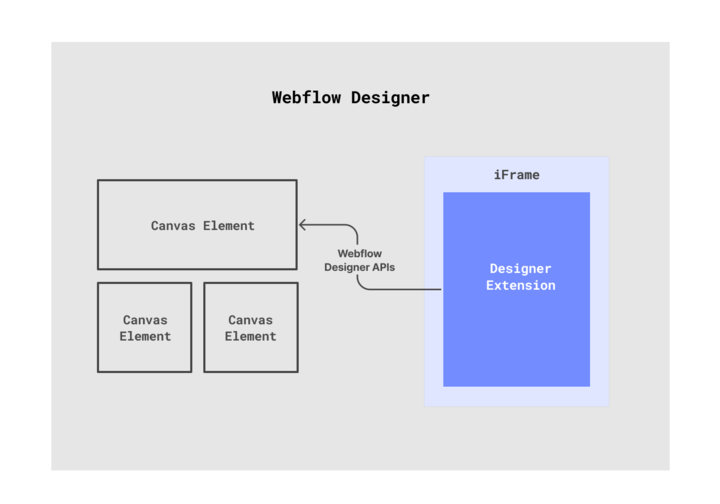
The Webflow CLI
To access the Designer APIs, you’ll need to download and install Webflow’s CLI from NPM. Using the CLI, you’ll be able to create a starter project that automatically creates the basic structure, files, and code for your Designer Extension, which include the Objects and Methods of the Designer API that will allow you to manipulate elements on the Webflow Canvas. Learn more about the Webflow CLI in the Designer API Reference.
Download the CLI
npm install -g @webflow/webflow-cli
Initialize your project
webflow extension init your-extension-name
When you initialize your project with the Webflow CLI, we provide some example files that include a header, main content area, and footer for a basic web app. You can get started with an example project by typing the following command, and replacing it with your Designer Extension’s name.
Review the starter code
Let’s walk through the key files needed to bring structure, style, and dynamic content to your apps. You can always learn more about an App’s configuration, structure, and necessary files in our App Structure docs.
App Structure | index.html | This file serves as the initial point of entry for your single page web app. It contains the basic structure and layout, acting as a "skeleton" that remains constant as users navigate through different sections of your app. Instead of loading complete new HTML pages, fetch data from APIs and use JavaScript to update the content dynamically within the existing index.html structure. |
CSS Styling | styles.css | This file defines the visual appearance of your App. It's responsible for determining how elements are positioned, their colors, typography, spacing, and overall design. styles.css plays a significant role in maintaining a consistent and appealing user interface across different views without requiring full page reloads. |
Dynamic Content | index.js / index.ts | This file adds interactivity and dynamic behavior to your web app. It's responsible for handling user interactions, fetching data from APIs, updating the DOM, and managing the flow of your application. index.js is central to creating the seamless user experience by enabling content updates without full page reloads. |
As you explore and modify the scaffold, don't be afraid to experiment and make changes. The best way to learn is by doing, and the scaffold is a safe environment to try things out.
Interacting with the Designer
Designer extensions elevate App functionality by directly interacting with elements on the Canvas. Let’s explore how you can use a Designer Extension and our Designer APIs to create, modify, and delete elements on an active Canvas.
Accessing the Designer APIs
Our Designer APIs interact with the canvas via user-friendly objects and methods via The Webflow Object. With these APIs you're able to interact with existing elements, or add new elements to the canvas.
Interacting with existing elements
Interacting with elements on the canvas typically involves three steps: referencing, staging, and syncing.
1. Getting a reference to an element | 2. Staging changes to an element | 3. Syncing changes back to the Designer |
---|---|---|
You can get a reference to an element on the canvas by calling webflow.getSelectedElement() . This method returns a Promise that resolves to the currently selected element. |
Once you have a reference to an element, you can stage changes to it using various methods on the element object. For example, you can set a custom attribute on the element using setCustomAttribute() |
After staging your changes, you can sync them back to the Designer by calling save() on the element |
const el = await webflow.getSelectedElement(); |
el.setCustomAttribute("my-attribute", "my-value"); |
javascript el.save(); |
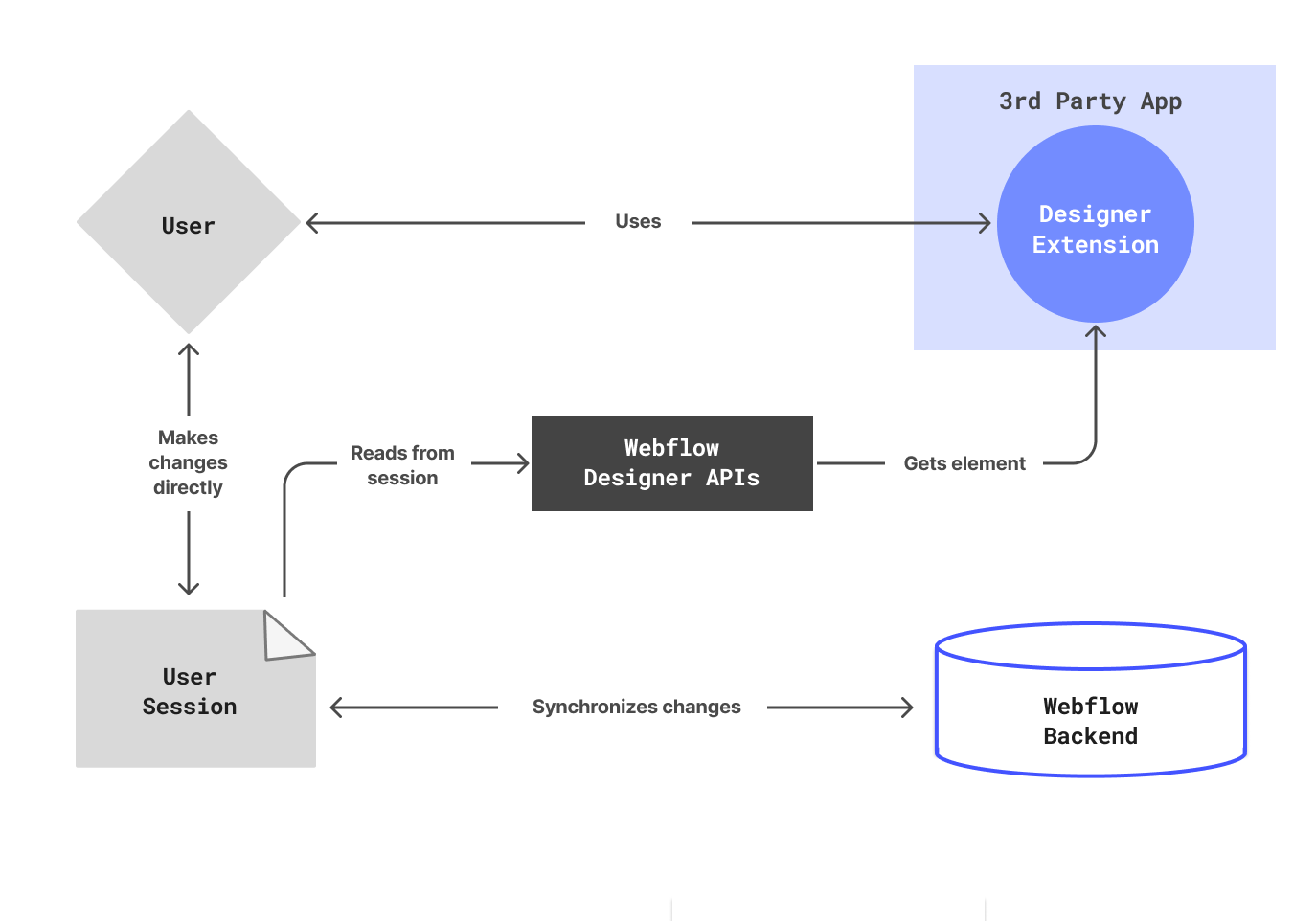
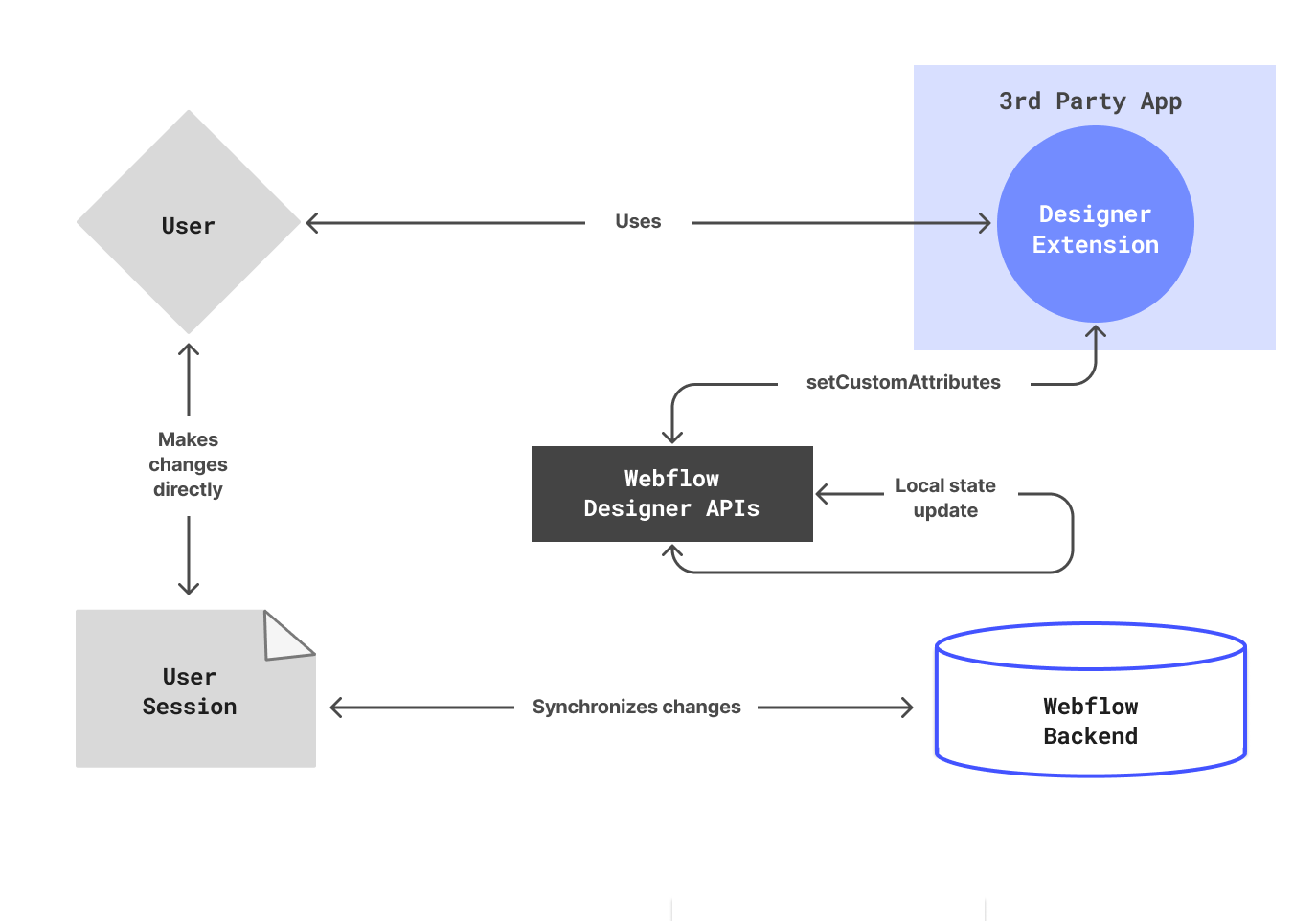
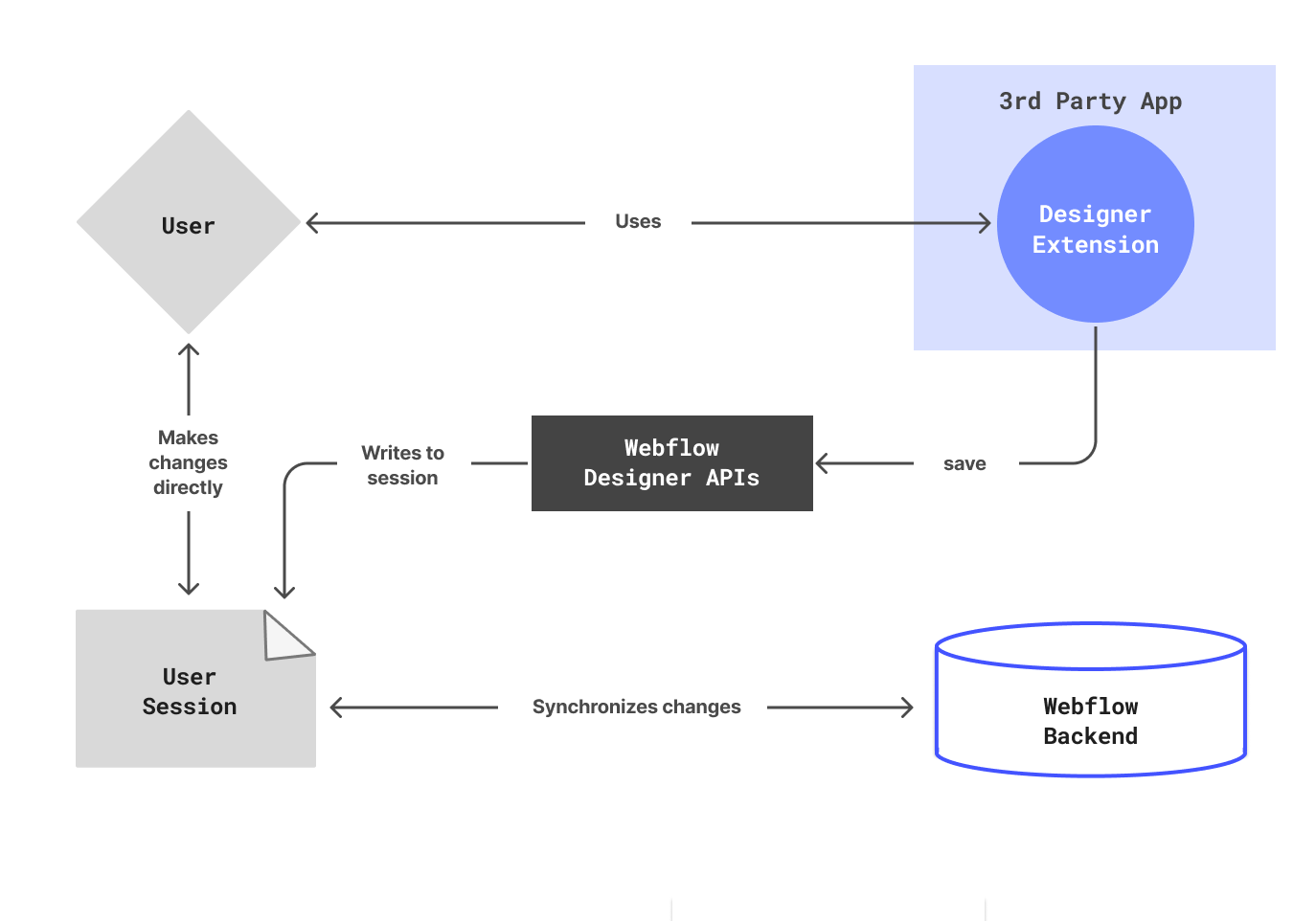
Creating new elements
In addition to manipulating existing elements, you may want to give your users the ability to add new elements to the Canvas. To do this, you can call the createDOM
method and pass the HTML tag of the type of element you’d like to create.
For example, you can create a new div
element with the following code:
const newDiv = webflow.createDOM("div");
You can then stage changes to this new element and sync them back to the Designer, just like with existing elements.
By understanding and using these methods, you'll be able to create powerful and interactive Designer Extensions that can enhance the Webflow experience for users.
Updated 20 days ago