Learn how to Create and Edit Components using Webflow APIs
Webflow Components are customizable blocks created from Elements. They serve as the backbone for structuring visual hierarchies on a Webflow site, ensuring that designs are modular, reusable, and consistent.
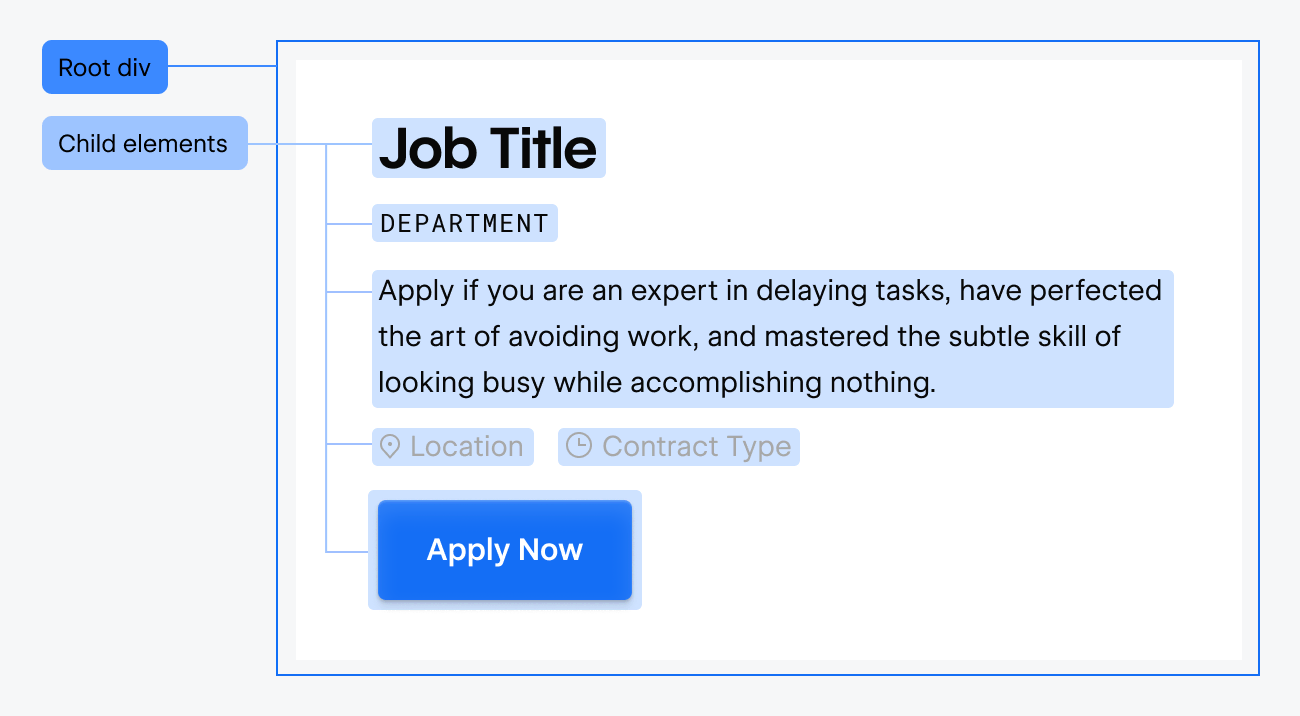
Let’s review some key concepts of Components, and how they’re implemented in Webflow.
Definitions
Component Definition
Also known as the component object, the component definition is the blueprint for a component. It establishes the foundational structure of the elements within the component, as well as properties that can be further defined in a component instance. Any modifications made to the component definition will propagate and automatically update all associated component instances. This ensures consistency across instances while allowing for centralized changes.
Use components to manage recurring layouts and content more efficiently across your site.
Component Instance
A component instance is a concrete instantiation of the component definition. While it retains the core design and structure of the definition, each instance can be customized through unique properties. This allows designers and users to assign custom values to a component instance’s properties, tailoring its appearance and behavior, without modifying the underlying component definition..
Component Operations
With Component concepts explained, here is a quick overview of the capabilities offered by the Components API:
🛠️ Create a Component Definition
To create a component definition you'll need a root element - or top-level element - that has child elements within its element hierarchy. With the root element identified, you can use webflow.registerComponent(rootElement)
to register the component definition. In the above card component example, the root element would be the container DIV element, which has the img
, div
, and button
child elements.
// Get selected element
const rootElement = await webflow.getSelectedElement();
if (rootElement) {
// Create a component from the Root Element
const component = await webflow.registerComponent('MyCustomComponent', rootElement);
console.log(`Component registered with ID: ${component.id}`);
} else {
console.log("No element is currently selected. Please select a root element first.");
}
✏️ Edit a Component Definition
- Get the component
- Get the root element of the component
- Insert / remove child elements from the root element.
// Get Component
const all = await webflow.getAllComponents()
const firstComponent = all[0]
// Get Root Element
const root = await firstComponent?.getRootElement() as AnyElement
if (root.children) {
// Append DIV block to Root element
await root?.append(webflow.elementPresets.DivBlock);
}
✏️ Adding a Component Instance to a Page
Use webflow.createInstance(componentDefinition)
to add a component instance to the page. Component instances can be nested as part of element hierarchies, including under other component instances.
Component Properties
Pre-defined attributes within a Component Definition that can be assigned a specific value in the Component Instance. They allow designers or users to modify specific aspects of an Instance - text, images, links, and more - without affecting its foundational design.
Component Properties
Currently our APIs do not yet support the creation and management of Component Properties.
We understand the importance of this feature for our users, and we're excited to share that it's on our development roadmap. We're committed to enhancing our API capabilities and will provide updates as we make progress. Thank you for your patience and understanding!