Elements
Element APIs allow you to create and manage Elements in the Designer. In this overview we’ll review the types of Elements you can insert onto the canvas, the methods that are particular to the properties or type of Element you’re working with, and the best practices for working with Elements.
Creating Elements
In order to add an Element into the Designer Canvas, you need to first select an Element Preset. Once selected, you can place a new Element on the canvas by either inserting it into a specific place in the existing Element Hierarchy, or by nesting elements inside of one another to create parent-child relationships.
Selecting an Element Preset
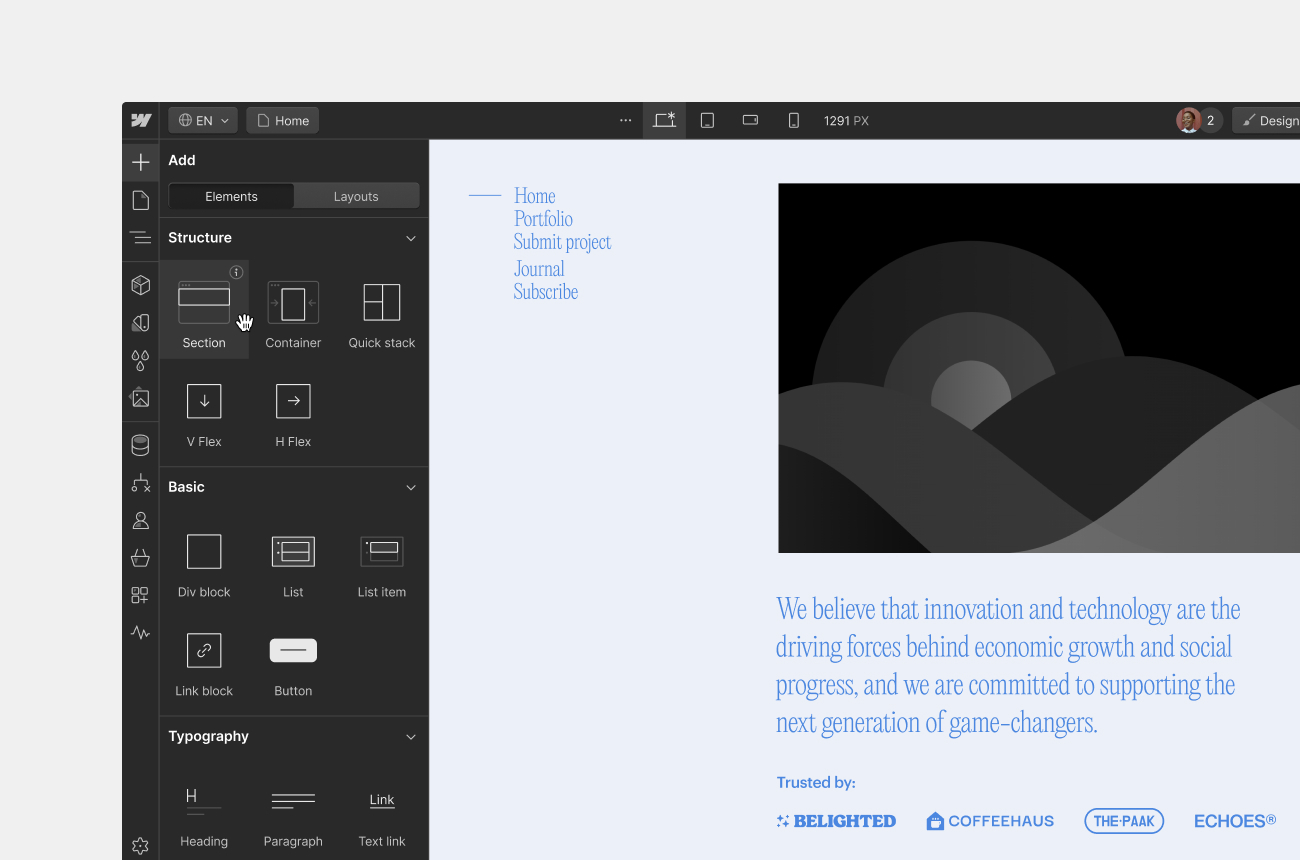
The Element Presets object specifies all the element types available in the Designer. Use the Element Presets object to select the Element type you’d like to insert onto a page.
With webflow.elementPresets.<preset>
, any Element Preset can be passed as a parameter to the Element creation methods as shown in the example below.
Once an element is created on the canvas, it can be manipulated through its respective methods, which we’ll cover in the sections below. Read through the full list of Element Presets to see all the Elements you can add to the canvas via the Designer API.
Adding Elements to the Canvas
Once you’ve identified the Element Preset you’d like to use, you can add it to the canvas via the Element creation methods. These methods allow you to place the Element on the canvas within a specific order of the Element Hierarchy, or nest elements inside of one another to create parent-child relationships.
Inserting an element
When inserting an element on the canvas, it’s important to consider the Element Hierarchy. To achieve the desired styling and flow, you need to understand where in the hierarchy your new element belongs. To insert an Element into the existing Element Hierarchy:
- Select an element using
webflow.getSelectedElement()
- Decide where your new Element will be positioned within the existing Element hierarchy by using:
before()
- this method will insert your new element before the selected elementafter()
- this method will insert your new element after the selected element
Note: If the selected element has a parent element, the new element created using before()
or after()
will also be a child element of the same parent.
Nesting a child element
Additionally, you can decide whether to establish parent-child relationships by nesting your element within another, which can greatly influence the layout and functionality of your design. In order to nest one element within another to create a parent-child relationship:
- Check that the element you’ve selected supports child elements, then
- Decide how the new Element will be nested within the selected element by using:
prepend()
- this method will insert your new Element as the first child elementappend()
- this method will insert your new Element as the last child element
Element Properties & Methods
Once an element is added or selected, a suite of Element methods are available to help you incorporate content, styles, and HTML attributes into your design. Elements have two distinct types of methods: property methods and element-specific methods.
Property Methods
An element’s properties define how you can manipulate the element on the canvas. Each type of element shares common properties that inform their respective methods. For more detailed information about specific methods for each property, refer to the ‘Element Properties & Methods’ section of the documentation.
Element Properties
Element-Specific Methods
Certain elements also possess methods unique to their type. You can identify an element’s type through the element.type
property. These types inherit the methods of their properties but also add additional functionalities.
For instance, a String Element has an element.textContent
property set to false
, meaning you cannot use the element.setTextContent()
method on it. However, the String Element does offer a unique method, element.setText()
, which lets you modify that element’s content. It’s crucial to always consider an element’s property and element methods to understand the available functionalities.
Best Practices for Working with Elements
Type Checking
Ensure you’re working with the correct element type to apply appropriate methods effectively. Confirm the element’s type before performing operations to prevent errors and achieve desired outcomes.