Styles are key to creating dynamic and responsive designs in Webflow.
In the Designer, you can manage style changes through Classes. In the Webflow API, these Classes are Style Objects - with distinct CSS properties - that can be applied to an element. The methods described below give you direct control over which styles are applied to elements, letting you change a page's appearance and layout as needed. To directly manage CSS properties within a Style Object, use the Style Methods.
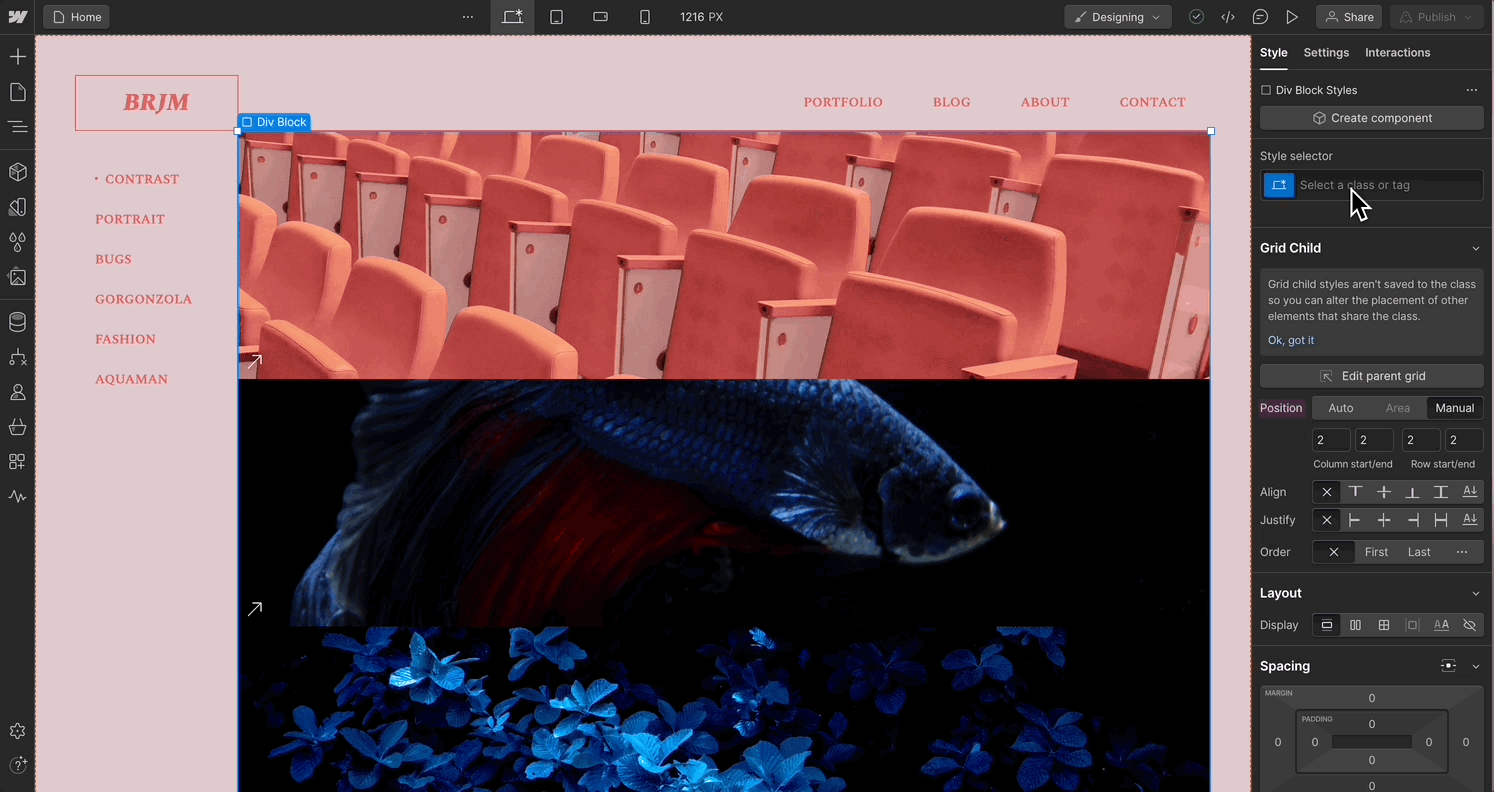
Methods
element.getStyles()
element.getStyles()
Retrieve the current style properties of the element for analysis or changes.
Syntax
element.getStyles(): Promise<Array<Style>>
Returns
Promise<Style>
A Promise that resolves to an array of Style Objects.
Example
// Get Selected Element
const selectedElement = await webflow.getSelectedElement()
if (selectedElement?.styles) {
// Get Styles
const styles = await selectedElement.getStyles()
// Get Style Details
const styleDetails = styles.map(async style => {
const styleName = await style.getName()
const styleProperties = await style.getProperties()
return {
Name: styleName,
Properties: styleProperties,
ID: style.id,
}
})
// Print Style Details
console.log(await Promise.all(styleDetails))
Designer Ability
Checks for authorization only
Designer Ability | Locale | Branch | Workflow | Sitemode |
---|---|---|---|---|
canAccessCanvas | Any | Any | Any | Any |
element.setStyles(styles)
element.setStyles(styles)
Set styles on an element.
Syntax
element.setStyles(styles: Array<Style>): Promise<null>>
Parameters
Styles
: Array of Style Objects - The array of styles to set.
Returns
Promise<null
>
A Promise that resolves to null
.
Example
// Get Selected Element
const selectedElement = await webflow.getSelectedElement()
if (selectedElement?.styles) {
// Create a new style
const newStyle = await webflow.createStyle("MyCustomStyle");
// Set properties for the style
newStyle.setProperties({
'background-color': "blue",
'font-size': "32px",
'font-weight': "bold",
});
// Set style on selected element
selectedElement.setStyles([newStyle])
}