Forms
Webflow Forms enable users to capture and collect information from visitors on a site.
Creating a form
Use the FormForm
element preset to create a form.
When you create a form using the FormForm
preset, it automatically generates a complete form structure with default form fields, as well as a success and error message.
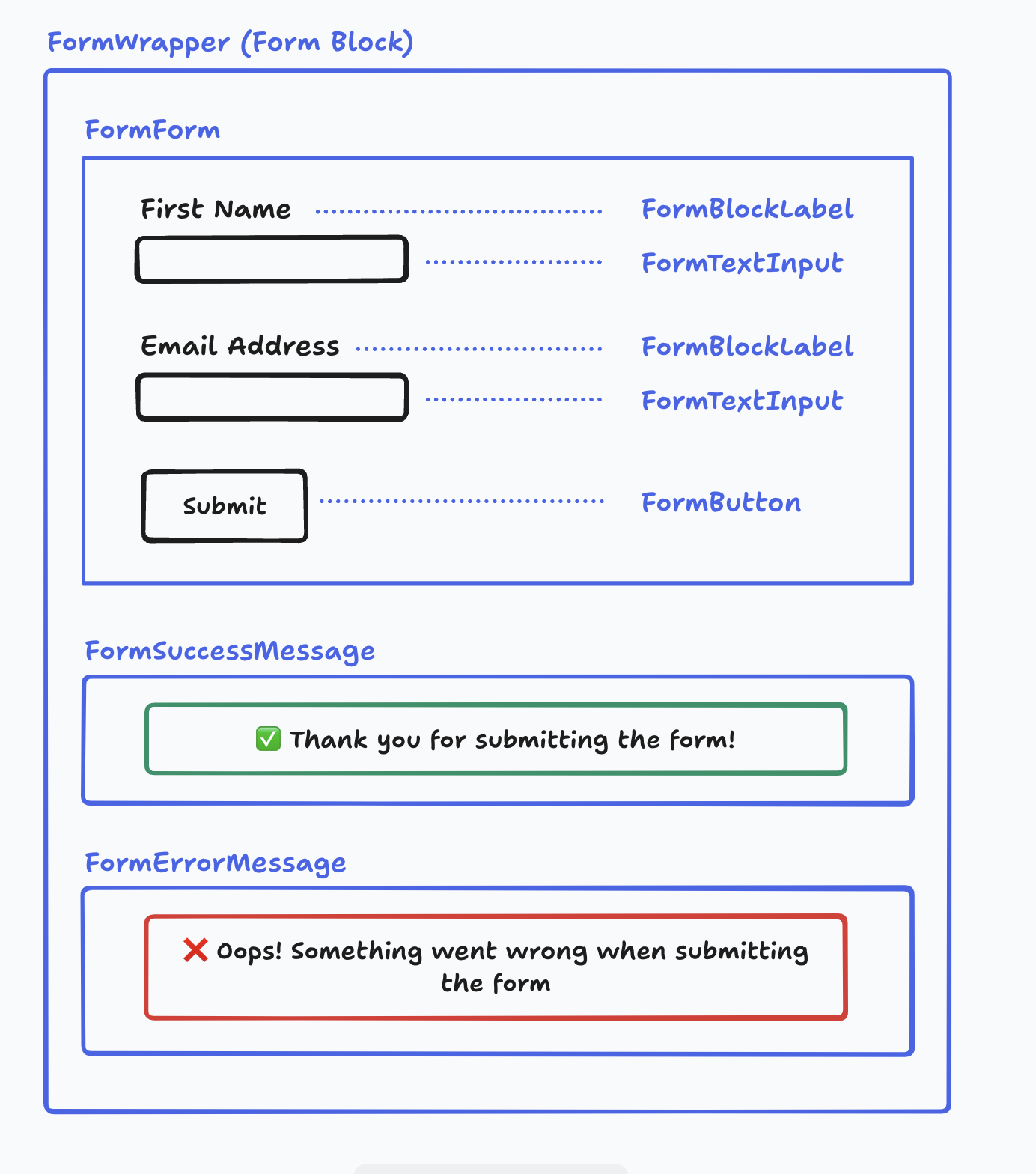
Form structure
A Webflow form consists of several nested elements that work together:
Form inputs
Form inputs are the individual fields that collect user information. It’s recommended to create a wrapper to contain each input and it’s corresponding label.
You can create form inputs using the following element presets:
FormTextInput
FormTextarea
FormSelect
FormCheckboxInput
FormRadioInput
Example
Form labels
Each input should have a label that describes the information it collects. Create a label using the FormBlockLabel
element preset to label each input.
Methods
Form element methods
FormForm
and FormWrapper
elements.The Form Element supports the following specific methods:
Retrieves the name of the form.
Sets the name of the form.
Retrieves the settings of the form.
Sets the settings of the form.
Form input methods
The following methods apply to form input elements:
Retrieves the required status of a form input.
Sets the required status of a form input.
Retrieves the name of the input field.
Sets the name of the input field.
Retrieves the type of the input field.
Sets the type of the input field.